[Python] 텍스트 파일 쓰기와 읽기 (writelines() 메소드, readlines() 메소드)
Python 분석과 프로그래밍/Python 설치 및 기본 사용법 2020. 3. 8. 22:36지난번 포스팅에서는 파일을 열고(open), 파일을 읽고(read), 쓰고(write), 닫기(close)를 하는 방법을 소개하였습니다.
이번 포스팅에서는 Python 에서
(1) 문자열로 이루어진 리스트를 텍스트 파일에 쓰기
- (1-1) 한 줄씩 쓰기 : write() 메소드 (for loop 문과 함께 사용)
- (1-2) 한꺼번에 모든 줄 쓰기 : writelines() 메소드
(2) 텍스트 파일을 읽어오기
- (2-1) 텍스트를 한 줄씩 읽어오기 : readline() 메소드 (while 문과 함께 사용)
- (2-2) 텍스트를 한꺼번에 모두 읽어오기 : readlines() 메소드
에 대해서 소개하겠습니다.
(1-1) 문자열로 이루어진 리스트를 한 줄씩 텍스트 파일에 쓰기 : write() 메소드 |
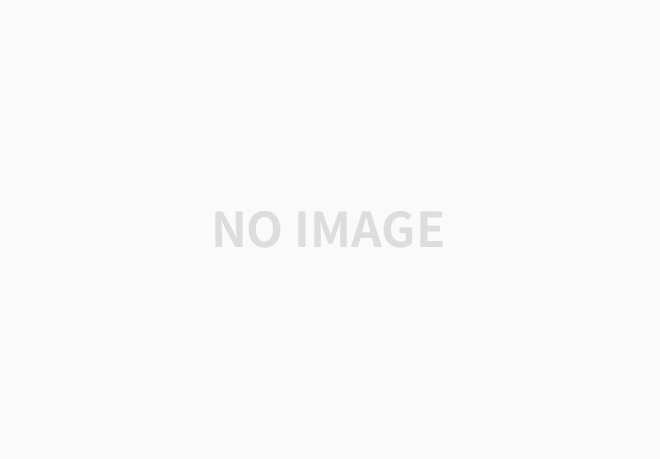
예제로 사용할 텍스트는 영화 매트릭스(movie Matrix)에서 모피어스가 네오에게 말했던 대사 (Morpheus Quotes to Neo) 중 몇 개로 이루어진 파이썬 리스트입니다. '\n'으로 줄을 구분하였습니다.
matrix_quotes = ["The Matrix is the world that has been pulled over your eyes to blind you from the truth.\n", "You have to let it all go, Neo. Fear, doubt, and disblief. Free your mind.\n", "There is a difference between knowing the path and walking the path.\n", "Welcom to the desert of the real!\n"]
|
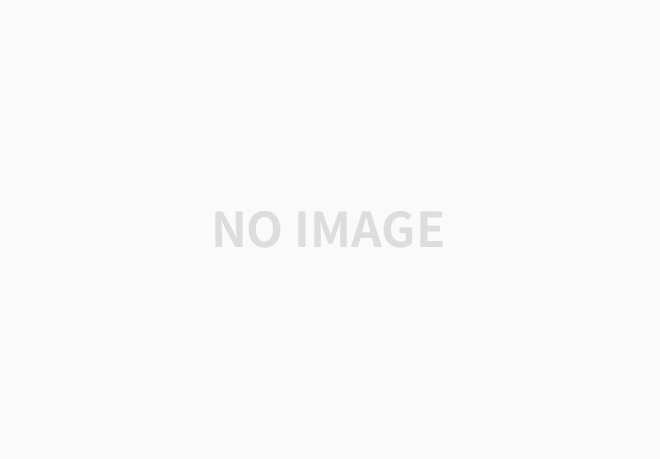
* image source: https://www.elitecolumn.com/morpheus-the-matrix-quotes/morpheus-the-matrix-quotes-4/
위의 텍스트 문자열로 이루어진 'matrix_quotes' 리스트를 'matrix_quotes.txt'라는 이름의 파일에 한 줄씩 써보도록 하겠습니다. 먼저 with open('matrix_quotes.txt', 'w') 함수를 사용해서 'matrix_quotes.txt'라는 이름의 파일을 '쓰기 모드('w')'로 열고, 모두 4줄로 이루어진 리스트이므로 for loop 순환문을 이용해서 한줄 씩 line 객체로 가져온 다음에 f.write(line) 메소드로 한 줄씩(line by line) 'matrix_quotes.txt' 텍스트 파일에 써보겠습니다.
with open('matrix_quotes.txt', 'w') as f: for line in matrix_quotes: f.write(line)
|
* 참고로, with open() 함수를 사용하면 close() 를 해주지 않아도 알아서 자동으로 close()를 해줍니다.
* with open('matrix_quotes.txt', 'w') 에서 'w' 는 쓰기 모드를 의미합니다.
현재 Jupyter notebook을 열어서 작업하고 있는 폴더에 'matrix_quotes.txt' 텍스트 파일이 생성되었음을 확인할 수 있습니다.
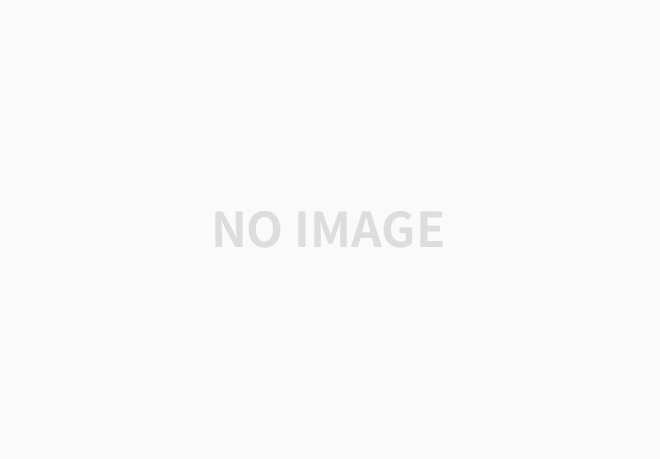
for loop 문과 write() 메소드를 사용해서 텍스트를 파일에 쓴 'matrix_quotes.txt' 파일을 더블 클릭해서 열어보니 Python list 의 내용과 동일하고, 줄 구분도 '\n'에 맞게 잘 써진 것을 알 수 있습니다.
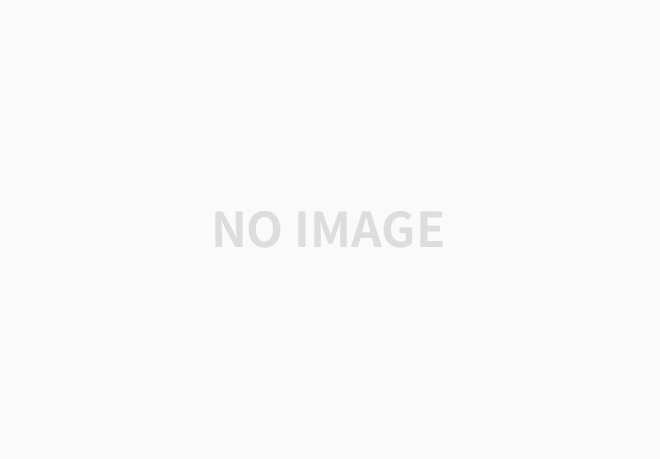
(1-2) 문자열로 이루어진 리스트의 모든 줄을 텍스트 파일에 쓰기: writelines() 메소드 |
그런데 위의 write() 메소드의 경우 여러 개의 줄이 있을 경우 for loop 반복 순환문을 함께 써줘야 하는 불편함이 있습니다. 이때 writelines() 메소드를 사용하면 리스트 안의 모든 줄을 한꺼번에 텍스트 파일에 써줄 수 있어서 편리합니다. 위의 (1-1)과 동일한 작업을 writelines() 메소드를 사용해서 하면 아래와 같습니다.
matrix_quotes = ["The Matrix is the world that has been pulled over your eyes to blind you from the truth.\n", "You have to let it all go, Neo. Fear, doubt, and disblief. Free your mind.\n", "There is a difference between knowing the path and walking the path.\n", "Welcom to the desert of the real!\n"]
with open('maxtix_quotes_2.txt', 'w') as f: f.writelines(matrix_quotes)
|
Jupyter notebook을 작업하고 있는 폴더에 가서 보니 'matrix_quotes_2.txt' 파일이 새로 잘 생성되었습니다.
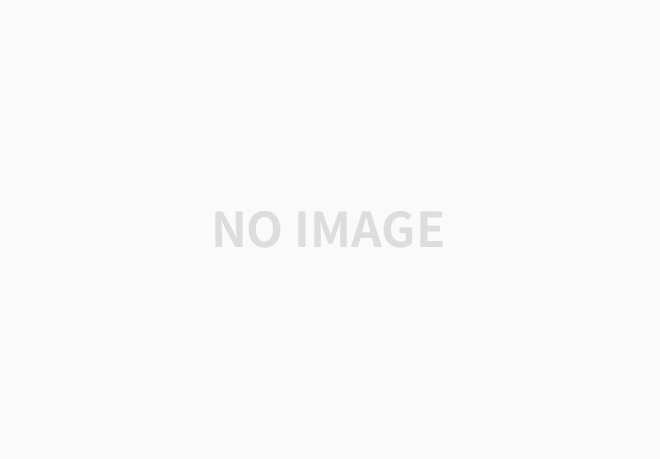
(2-1) 텍스트 파일을 한 줄씩 읽어오기 : readline() 메소드 |
이제는 방금전 (1-1)에서 만든 'matrix_quotes.txt' 텍스트 파일로 부터 텍스트를 한 줄씩 읽어와서 matrix_quotes_list 라는 이름의 파이썬 리스트를 만들어보겠습니다.
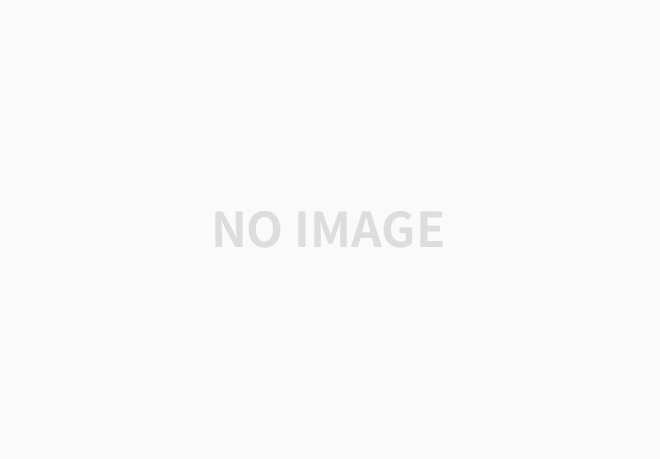
readline() 메소드는 텍스트 파일을 '\n', '\r', '\r\n' 과 같은 개행 문자(newline) 를 기준으로 한 줄씩 읽어오는 반면에 vs. readlines() 메소드는 텍스트 파일 안의 모든 텍스트를 한꺼번에 읽어오는 차이가 있습니다. 따라서 한 줄씩만 읽어오는 readline() 메소드를 사용해서 텍스트 파일 내 모든 줄을 읽어오려면 while 순환문을 같이 사용해주면 됩니다.
with open('matrix_quotes.txt', 'r') as text_file: matrix_quotes_list = [] line = text_file.readline() while line != '': matrix_quotes_list.append(line) line = text_file.readline()
|
matrix_quotes_list
|
* 참고로 with open('matrix_quotes.txt', 'r') 에서 'r'은 '읽기 모드'를 의미합니다.
만약 파일을 한 줄씩 읽어오면서 매 10번째 행마다 새로운 파일에 쓰기를 하고 싶다면 아래 코드 참고하세요.
with open(in_name, 'r') as in_file:
with open(out_name, 'w') as out_file:
count = 0
for line in in_file:
if count % 10 == 0:
out_file.write(line)
count += 1
(2-2) 텍스트 파일을 모두 한꺼번에 읽어오기 : readlines() 메소드 |
이번에는 readlines() 메소드를 사용해서 'matrix_quotes.txt' 텍스트 파일 안의 모든 줄을 한꺼번에 읽어와서 matrix_quotes_list_2 라는 이름의 파이썬 리스트를 만들어보겠습니다. readlines() 메소드는 한꺼번에 텍스트를 읽어오기 때문의 위의 (2-1) 의 readline()메소드와 while 순환문을 함께 쓴 것도다 코드가 간결합니다.
with open('matrix_quotes.txt', 'r') as text_file: matrix_quotes_list_2 = text_file.readlines()
matrix_quotes_list_2
|
많은 도움이 되었기를 바랍니다.
이번 포스팅이 도움이 되었다면 아래의 '공감~
'를 꾹 눌러주세요. :-)
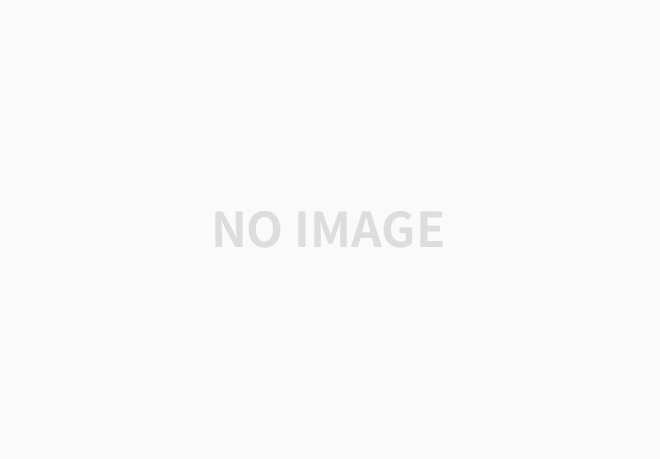
'Python 분석과 프로그래밍 > Python 설치 및 기본 사용법' 카테고리의 다른 글
Rfriend님의
글이 좋았다면 응원을 보내주세요!