[Python pandas] DataFrame의 칼럼 순서 바꾸기 (To change the order of DataFrame columns)
Python 분석과 프로그래밍/Python 데이터 전처리 2021. 9. 3. 21:11이전 포스팅에서는 Python pandas DataFrame의 칼럼 이름을 바꾸는 방법 (https://rfriend.tistory.com/468)을 소개하였습니다.
이번 포스팅에서는 pandas DataFrame의 칼럼 순서를 바꾸는 방법(how to change the order of pandas DataFrame columns?)을 소개하겠습니다.
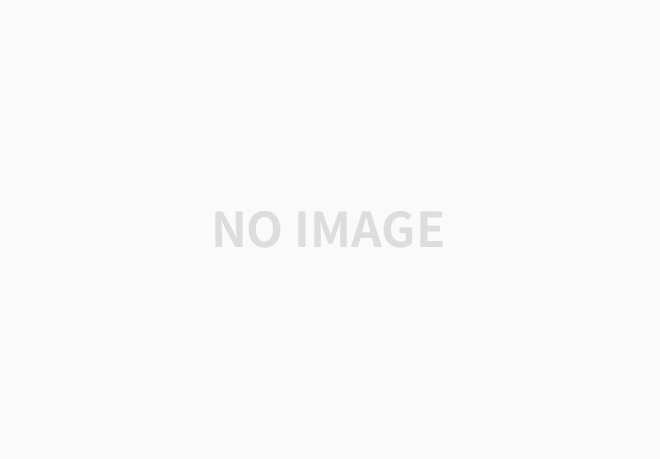
먼저 6개의 칼럼을 가진 간단한 pandas DataFrame 을 만들어보겠습니다.
import numpy as np
import pandas as pd
# making the sample DataFrame
df = pd.DataFrame(np.arange(12).reshape(2, 6),
columns=['x1', 'x2', 'x3', 'x4', 'x5', 'y'])
print(df)
# x1 x2 x3 x4 x5 y
# 0 0 1 2 3 4 5
# 1 6 7 8 9 10 11
위의 예제 DataFrame 'df'에서 마지막에 있는 칼럼 'y'를 제일 앞 위치로 변경을 해보겠습니다.
(1) 새로운 순서의 칼럼 이름 리스트로 인덱싱 해와서 새로운 DataFrame 만들기
# (1) reassigning the new order of columns
df2 = df[['y', 'x1', 'x2', 'x3', 'x4', 'x5']]
print(df2)
# y x1 x2 x3 x4 x5
# 0 5 0 1 2 3 4
# 1 11 6 7 8 9 10
(2) 칼럼 리스트의 마지막 위치(-1)로 인덱싱해서 새로운 순서의 칼럼 리스트 만든 후, 새로운 DataFrame 만들기
# (2) reassignning with a list of columns
col_list = df.columns.tolist()
print(col_list)
#['x1', 'x2', 'x3', 'x4', 'x5', 'y']
new_col_list = col_list[-1:] + col_list[:-1]
print(new_col_list)
#['y', 'id', 'x1', 'x2', 'x3', 'x4', 'x5']
df3 = df[new_col_list]
print(df3)
# y x1 x2 x3 x4 x5
# 0 5 0 1 2 3 4
# 1 11 6 7 8 9 10
(3) 문자와 숫자로 구성된 여러개의 칼럼 이름을 for loop 순환문으로 만들어서 새로운 순서의 칼럼 리스트를 만든 후, 새로운 DataFrame 만들기
## (3) making columns list using concatenation and for-loop
new_col_list2 = ['y'] + ['x'+str(i+1) for i in range(5)]
print(new_col_list2)
#['y', 'x1', 'x2', 'x3', 'x4', 'x5']
print(df[new_col_list2])
# y x1 x2 x3 x4 x5
# 0 5 0 1 2 3 4
# 1 11 6 7 8 9 10
(4) 숫자 위치 인덱스를 사용해서 새로운 순서로 칼럼을 인덱싱해와서, 새로운 DataFrame 만들기
# (4) reassignning the order using Positioning index
df4 = df.iloc[:, [5, 0, 1, 2, 3, 4]]
print(df4)
# y x1 x2 x3 x4 x5
# 0 5 0 1 2 3 4
# 1 11 6 7 8 9 10
(5) 칼럼 이름이 'y' 이 것과 'y'가 아닌 조건문(if condition statement)으로 새로운 순서의 칼럼 라스트를 만들어서, 새로운 DataFrame 만들기
# (5) reassignning the column order using condition statement
df5 = df[['y'] + [col for col in df.columns if col != 'y']]
print(df5)
# y x1 x2 x3 x4 x5
# 0 5 0 1 2 3 4
# 1 11 6 7 8 9 10
이번 포스팅이 많은 도움이 되었기를 바랍니다.
행복한 데이터 과학자 되세요! :-)
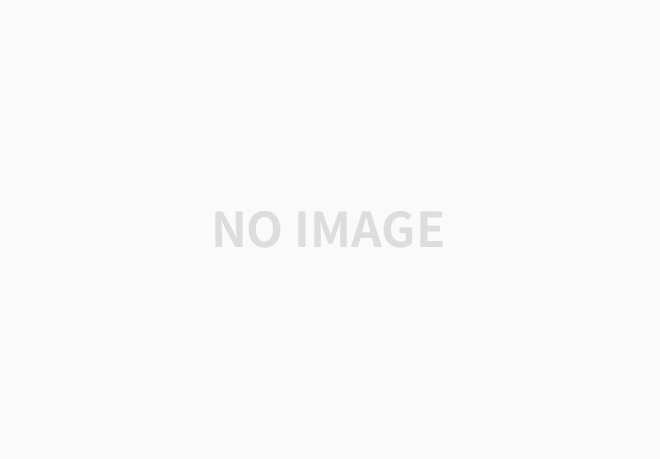
'Python 분석과 프로그래밍 > Python 데이터 전처리' 카테고리의 다른 글
Rfriend님의
글이 좋았다면 응원을 보내주세요!